Schedule Apex & Schedule Flow
What is Apex Scheduler?
Salesforce schedules (delay execution) the Apex class for execution at the specified time to run using Apex Scheduler. To take an advantage of Apex Scheduler, first we must write Apex class with Schedulable interface. Apex Scheduler invokes the Apex class to run at specific time. Anybody who want to schedule the schedule their class, they must have to implement schedulable interface.
Schedulable Interface ?
The class the implements this interface can be scheduled to run at different intervals. This Scheduled interface has several methods they are
public void execute(SchedulableContext SC)
Example
public class MySchedule implements schedule {
public void execute(ScheduableContext SC) {
Opportunity opp =new Opportunity (Name='Prasanth');
insert a;
}
}
How to implement Apex Scheduler ?
To implement Apex Scheduler follow the steps given below.
- Create an object for the class which has implemented the schedulable interface.
- Create the time frame.
- Invoke the System.Schedule method with job name, schedule object and time frame.
System.Schedule() method.
RemindOpptyOwners reminder = new RemindOpptyOwners();
// Seconds Minutes Hours Day_of_month Month Day_of_week optional_year
String sch = ’20 30 8 10 2 ?’;
String jobID = System.schedule(‘Remind Opp Owners’, sch, reminder);
As shown above, the expression is written in the form of “Seconds, minutes, hours, day of month, month, day of the week, optional year”.
Seconds | Minutes | Hours | Day-Month | Month | Day-week | Optional year |
20 | 30 | 8 | 10 | 2 | 0 | 2017 |
Apex Scheduler will run in system context, which means all the classes are executed whether the user has permission or not. We can monitor and stop the execution of Apex scheduler job using Salesforce user Interface from setup.
- Navigate to Setup | Apex Class | Click Schedule Apex.
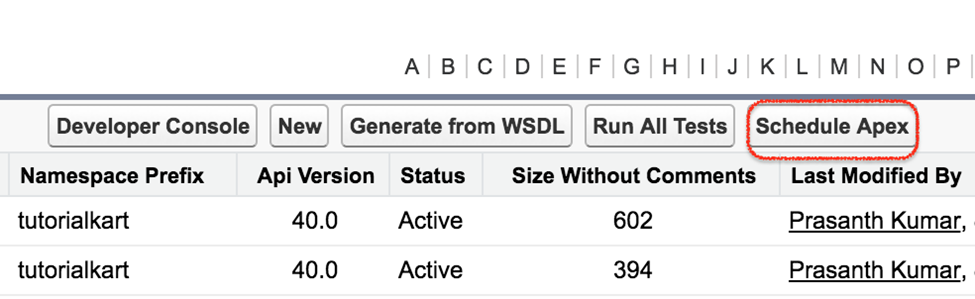
- Enter Job name and select Apex class from the lookup.
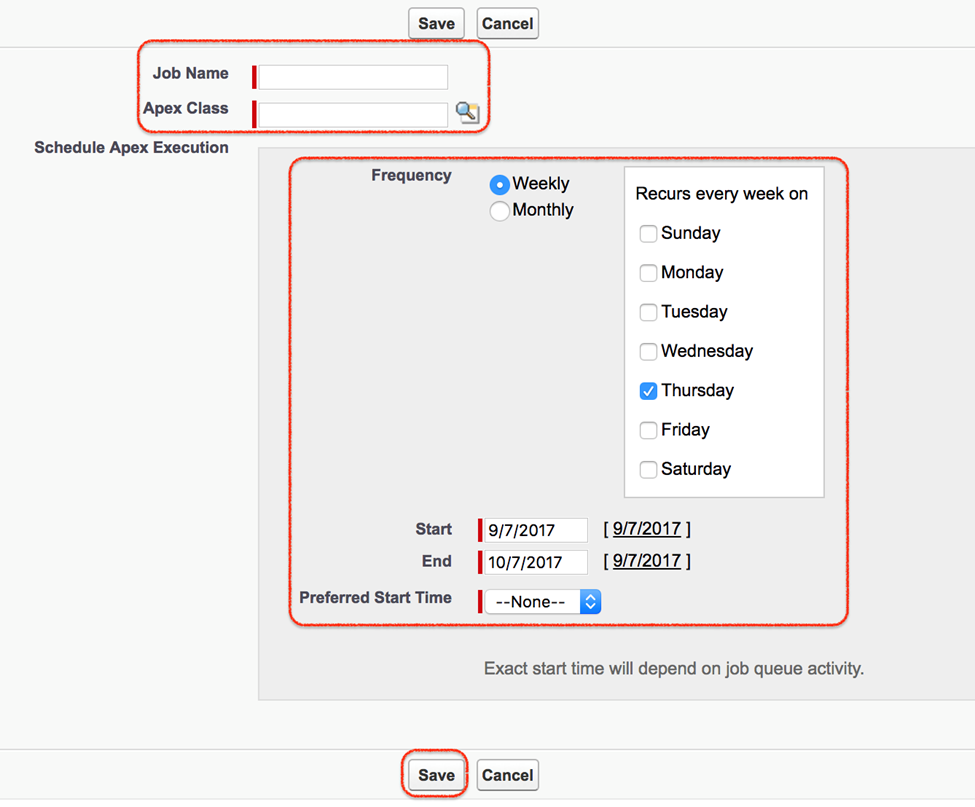
- Select Weekly or Monthly for the frequency and set the frequency desired.
- Select the start and end dates, and a preferred start time.
- Click Save.
Test Schedule Apex :
@isTest
private class RemindOppyOwnersTest {
// Dummy CRON expression: midnight on March 15.
// Because this is a test, job executes
// immediately after Test.stopTest().
public static String CRON_EXP = '0 0 0 15 3 ? 2022';
static testmethod void testScheduledJob() {
// Create some out of date Opportunity records
List<Opportunity> opptys = new List<Opportunity>();
Date closeDate = Date.today().addDays(-7);
for (Integer i=0; i<10; i++) {
Opportunity o = new Opportunity(
Name = 'Opportunity ' + i,
CloseDate = closeDate,
StageName = 'Prospecting'
);
opptys.add(o);
}
insert opptys;
// Get the IDs of the opportunities we just inserted
Map<Id, Opportunity> opptyMap = new Map<Id, Opportunity>(opptys);
List<Id> opptyIds = new List<Id>(opptyMap.keySet());
Test.startTest();
// Schedule the test job
String jobId = System.schedule('ScheduledApexTest',
CRON_EXP,
new RemindOpptyOwners());
// Verify the scheduled job has not run yet.
List<Task> lt = [SELECT Id
FROM Task
WHERE WhatId IN :opptyIds];
System.assertEquals(0, lt.size(), 'Tasks exist before job has run');
// Stopping the test will run the job synchronously
Test.stopTest();
// Now that the scheduled job has executed,
// check that our tasks were created
lt = [SELECT Id
FROM Task
WHERE WhatId IN :opptyIds];
System.assertEquals(opptyIds.size(),
lt.size(),
'Tasks were not created');
}
}
Schedulable Apex Limitations:
- We can schedule only 100 jobs at a time.
- Maximum number of Apex schedule jobs in 24 hours is 2,50,000.
- Synchronous Web service callouts are not supported in schedulable Apex.
Difference Between Schedule Apex and Schedule Flow
Schedule Flow | Schedule Apex |
Scheduled flows are essentially a low-code version of Scheduled Batch Apex | It is running a piece of apex code at some particular time within a period of time. |
It can only query upto 50,000 records in regular transaction | It Allows You to query upto 50 Million records |
What is Schedule Flow?
- A schedule-triggered flow starts at the specified time and frequency.
- And they run in background You can’t launch a schedule-triggered flow by any other means.
- The Start Time field value is based on the Salesforce org’s default time zone.
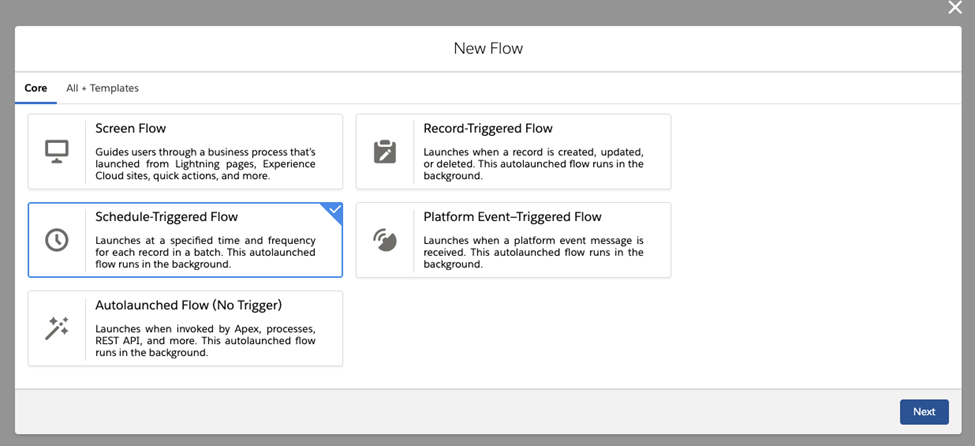
Next, Set a Schedule for your Flow by determining the following:
- Start Date: Determines the first time your Flow will run (be sure to set this in the future!) .
- Start Time: Determines the time of day your Flow will run.
- Frequency: Determines how often your Scheduled Flow repeats; in our example, we will set Frequency to “Weekly.”
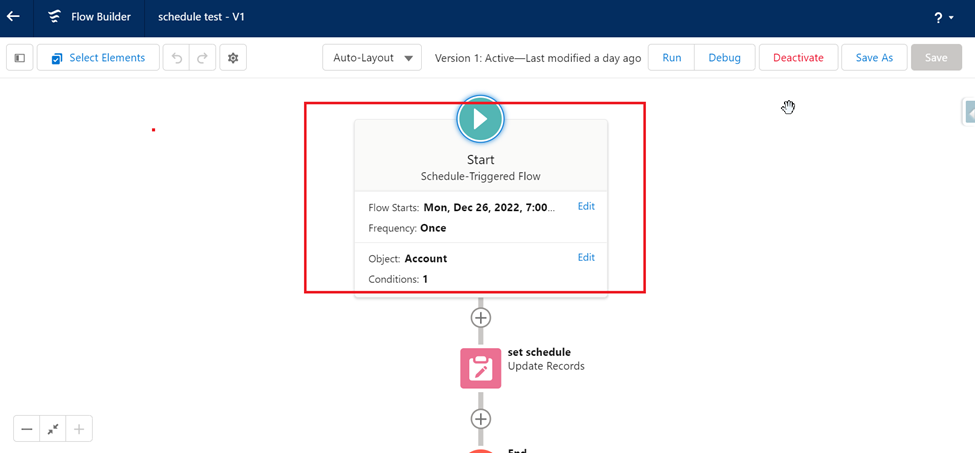
Next, We have to select object and give conditions to the particular field.
Next, we will create a update Records element to identify the source records, in our case, Account. You may set any conditional requirements .
Be sure to store all the records and all fields, as this will help you later if you decide to add fields to the Metric Object or grow the scope of your Scheduled Flow.
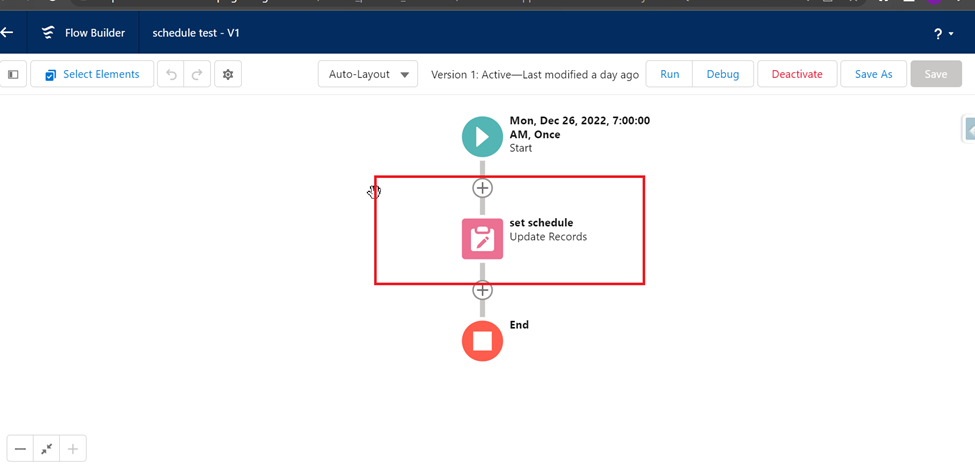
Query limit for Scheduled Flow:
- Scheduled flows are basically the low code replacement for Scheduled Batch Apex.
- In a normal transaction, you can only query up to 50k Records (Governor limit).
However, Batch Apex, allows you to query up 50 Million Records.